The task was:
We have a special device for converting numbers into images. Can you tell us which keys did we press?
with a firmware hex file (firmware.hex
), a display dump (display.dat
) and a picture of the circuit:
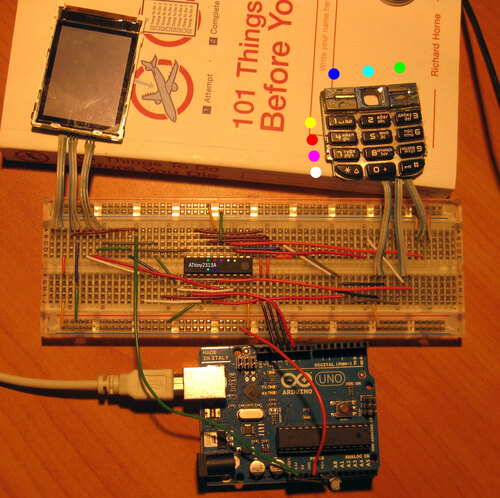
Finding main
The first part was finding the main loop of the firmware. Browsing through the code we found an very suspicious loop with only two function calls and some initializing stuff before.
1 2 3 4 5 6 7 8 9 10 |
|
Analyze the first function
After we found the main-loop we started analyzing the first function at 0x11f
.
Reading the assembly we saw multiple read and write operations on the I/O-pins of the keypad.
This was the get_key
function, which saves the pressed digit into r24
. If the key was the one with the asterisk or the hash or even no key was pressed it set r24
to 0xff
.
Analyze the second function
The second function (at 0x17c
)started working with the register r24
, so we were quite sure it was the key handler.
Trying to understand what this function does, we analyzed the functions called within this function.
So we revealed:
mul_8
at0x218
: performs multiplication on 8bit registers
r24 = r24 * r22
mul_16
at0x221
: performs multiplication on 16bit registers
r24:r25 = r24:r25 * r22:r23
div_mod
at0x223
: calculates modulo and quotient of two 16bit registers
r24:r25 = r24:r25 % r22:r23
r22:r23 = r24:r25 / r22:r23
div_mod2
at0x247
: calculates modulo and quotient of two 16bit registers, with some magic if the msb is set
r24:r25 = r24:r25 % r22:r23
r22:r23 = r24:r25 / r22:r23
send_data
at0x0a9
: calculatesn = (x1 - x0 + 1) * (y1 - y0 + 1)
and sends some PCF8833 like spi magic to writen
bytes on the display
x0 = r24
y0 = r22
x1 = r20
y1 = r18
data = r16
Knowing these functions, it is “very easy” to understand the handle_key
function:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 |
|
The program writes the pressed key (digit* 0x19
) at the n * 0x38 + 1
position and saves n * 0x19
at position 0.
Using the following python script are we able to reveal the pressed keys.
1 2 3 4 5 6 7 |
|
The flag was: 424986074